Process Control for Converting Animations to Video - Our JavaScript API
Html2Video uses a full-functional web browser to play your animations. In order to catch events of animation start and stop our API has to be either included or sideloaded to your animation.
This RFQ is a proposal for standardizing animation control for the Html2Video managed recording environment. Individual playback logic is supported by the internal state machine as long as the playback is linear (this is a restriction of the video format).
The API implemented and published here intends to standardize the controlling layer so that all internal implementations of playback functionality can be tested in a basic browser.
Download the minified version of the Html2Video JavaScript API here!
Overview
The following drawing illustrates the general overview of the control structure of Html2.Video conversion service.
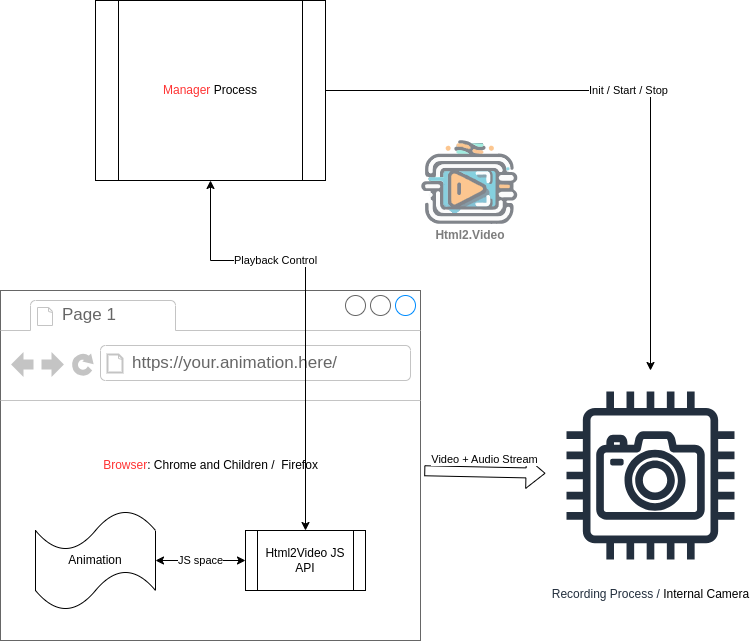
Controlling Playback via the Html2Video JS API
The Html2Video JS API will publish the following control handles. To achieve complience, the animation shall be properly connected to the hooks defined in this API.
Name | Command | Function / Description |
---|---|---|
Configuration | "HTML2VIDEO: option startstop=duration" | default, the Manager will force the duration timing on record independent of the video playback |
Configuration | "HTML2VIDEO: option startstop=stop" | the Manager will wait for the Stop Signal from the animation to stop recording |
Duration info | "HTML2VIDEO: option duration=12400" | duration in milliseconds: 12400 (12.4s) |
Generic Config options | "HTML2VIDEO: option key=value" | No special character in key (space, =) are allowed, value is free from this restriction |
Ready to Play | "HTML2VIDEO: Ready" | DOM document loaded (buffering, preload finished) |
Start Signal | "html2video._startAnimationCommand();" | commands the Animation to start playing (not public, but publicly accessable) |
Status Feedback Start Signal | "HTML2VIDEO: Started" | Playback successfully started |
Status Feedback Start Signal | "HTML2VIDEO: Failed" | Playback failed to commence |
Stop Signal | "HTML2VIDEO: Stop" | Playback finished, configuration dependently required |
Best practices for accurate timing
- preload (buffer) all media contents in the Browser, but do NOT start playing immediately after
- disable visual control elements (e.g. in a sideloaded JS)
- when using third party media content in-between steps, use precautious timing to assure proper buffering and allow time to play
Stop Contitions
The decision when to stop recording depends on the configuration. Available stop signals :
- Timer based: using value from JobDef.
- Timer based: using value from the variable read from Browser (Configuration, Duration).
- Trigger based: using the Stop Signal.
Definitons
- JobDefinition (JobDef): The parameters provided for processing an animation: [resolution, frame rate, duration, URL].
- Browser: Supervised browser running in our environment to interpret all html / JavaScript animation instructions.
- Animation: Animated content playable in a Browser.
- Manager Process (Manager): Custom software implementing controlling steps to get accurate playing / recording of the Animations.
- State Machine: The Manager Process can implement custom controlling logic in order to support different playback logic and signalling. State Machine describes the very logic it must follow by each playback session.
- Ready to Play (Ready Event): Information from the Animation to the Manager that the Animation can be played, and all caches and pre-buffers are properly filled.
- Stop Signal: Information from the Animation to the Manager that the Animation has finished, and recording can be stopped.
- Start Signal: Command from the Manager to the Animation, that the playing process shall begin.
Examples
General
Step | Description | Commands |
---|---|---|
1. | Size and FPS are defined in the JobDef. | |
2. | Browser started with the URL, and proper window size. | |
3. | When content is ready in the Browser, the Animation logs on JS Console | "HTML2VIDEO: option startstop=duration"; "HTML2VIDEO: option duration=12400"; |
4. | Browser reports Ready to Play | "HTML2VIDEO: Ready" |
5. | Manager instructs animation to start playback by calling the function | "html2video._startAnimationCommand();" |
6A | Manager stops recording exactly after 12400 ms | |
6B | OR - On playback finished, the Animation logs to JS Console | "HTML2VIDEO: Stop" |
Required interactions are marked RED in the following diagram:
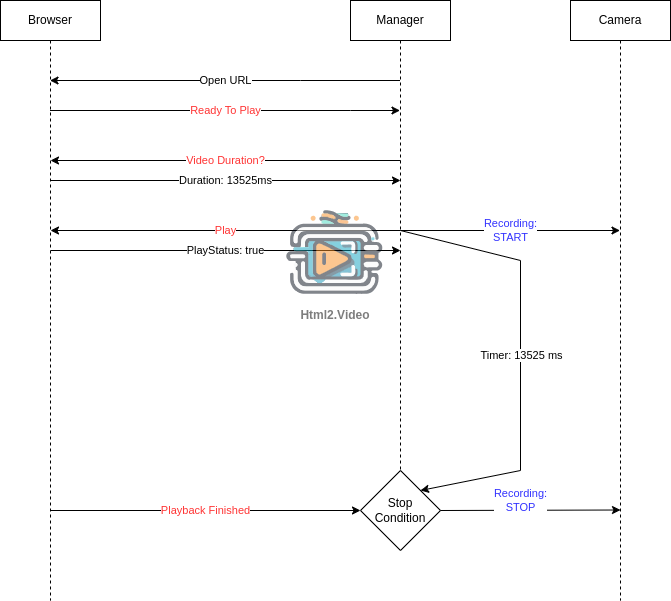
Asynchronous Control, Defining Duration
In the following example the duration of the animation is defined using the "duration" method for stopping the animation. To achive this the API is told that duration is to be taken account and the duration is 15 seconds. The signals are propagated asynchronously.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Title</title> <script type="text/javascript"> function onLoadedHtml2Video() { html2video.init({ stopMode: 'DURATION', duration: 15 * 1000 }); html2video.play(async () => { // wait something await new Promise((resolve) => setTimeout(() => resolve(), 3000)); // signal to start return true; }); } </script> <script type="text/javascript" src="html2video-latest.js" onload="onLoadedHtml2Video()" ></script> <script type="text/javascript"> // emulate backend setTimeout(() => { html2video._startAnimationCommand(); }, 1000); </script> </head> <body></body> </html>
Asynchronous Control, with Stop Signal
In this example the duration is kept in the JavaScript space, only the end of the animation is indicated using the "stop" command. To achieve this the API is told that STOP command is to be taken account. The signals are propagated asynchronously.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Title</title> <script type="text/javascript"> function onLoadedHtml2Video() { html2video.init({ stopMode: 'STOP' }); html2video.play(async () => { // wait something await new Promise((resolve) => setTimeout(() => resolve(), 3000)); // user start your player setTimeout(() => { // send signal is stop html2video.stop(); }, 3000); // signal to start return true; }); } </script> <script type="text/javascript" src="html2video-latest.js" onload="onLoadedHtml2Video()" ></script> <script type="text/javascript"> // emulate backend setTimeout(() => { html2video._startAnimationCommand(); }, 1000); </script> </head> <body></body> </html>
Example Control using Stop Signal
In this example the duration is kept in the JavaScript space, only the end of the animation is indicated using the "stop" command. To achieve this the API is told that STOP command is to be taken account.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <title>Title</title> <script type="text/javascript"> function onLoadedHtml2Video() { html2video.init({ stopMode: 'STOP' }); html2video.play(() => { // user start your player setTimeout(() => { // send signal is stop html2video.stop(); }, 3000); // signal to start return true; }); } </script> <script type="text/javascript" src="html2video-latest.js" onload="onLoadedHtml2Video()" ></script> <script type="text/javascript"> // emulate backend setTimeout(() => { html2video._startAnimationCommand(); }, 1000); </script> </head> <body></body> </html>
Questions?
We are here to help
Email Us
sales@html2.video
24/7 Technical Support
support@html2.video